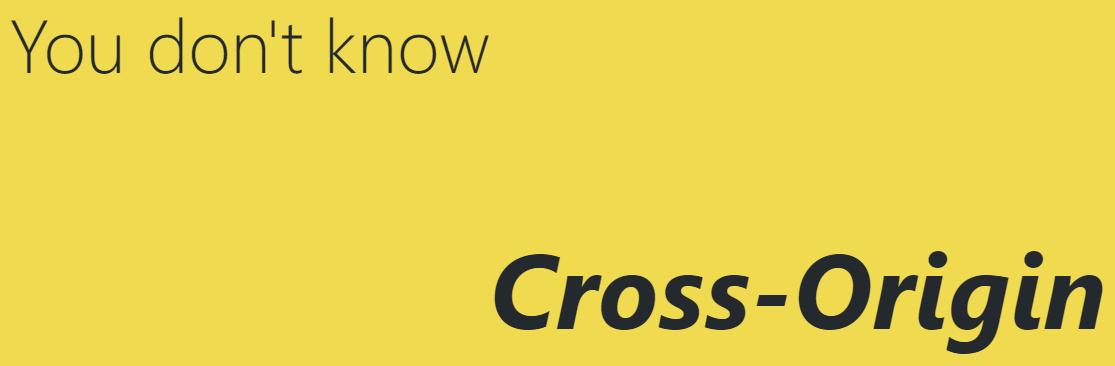
Why
为什么会存在跨域问题
- 同源策略
由于出于安全考虑,浏览器规定JavaScript不能操作其他域下的页面DOM,不能接受其他域下的xhr请求(不只是js,引用非同域下的字体文件,还有canvas引用非同域下的图片,也被同源策略所约束)
只要协议、域名、端口有一者不同,就被视为非同域。
How
如何解决
要解决跨域问题,就要绕过浏览器对js的限制,另辟蹊径
- JSONP
这是最简单,也是最流行的跨域解决方案,它利用script标签不受同源策略的影响,解决跨域,需要后台配合,返回特殊格式的数据
前端1
2
3
4
5
6
7
8
9
10
11
12
13<script>
function JSONP(link) {
let script=document.createElement("script");
script.src=link;
document.body.appendChild(script);
}
function getUser(data) {
console.log(data);// todo
}
const API_URL_USER='http://cache.video.iqiyi.com/jp/avlist/202861101/1/?callback=getUser'; // 这里以爱奇艺的接口为例(来源网络,侵删)
JSONP(API_URL_USER);
</script>
后端1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18// Express(Nodejs)
// mock data
const USERS=[
{name:"Tom",age:23},
{name:"Jack",age:23}
];
app.get("/user",function (req,res) {
let cbName=req.query["callback"];
// 这里做一个容错处理
res.send(`
try{
${cbName}(${JSON.stringify(USRES)});
}catch(ex) {
console.error("The data is invalid");
}
`);
});
- CORS (cross-origin resource sharing)
跨域资源共享,是W3C的一个标准,它允许浏览器发送跨域服务器的请求,CORS需要浏览器和服务器同时支持
后端
简单请求和非简单请求详情,请阅读阮一峰老师的博文,这里不再敖述1
2
3
4
5
6
7app.use(function (req,res,next){
res.header('Access-Control-Allow-Origin', 'http://localhost:6666'); // 允许跨域的白名单,一般不建议使用 * 号
res.header('Access-Control-Allow-Methods', 'GET,PUT,POST,DELETE'); // 允许请求的方法,非简单请求,会进行预检
res.header('Access-Control-Allow-Headers', 'Content-Type'); // 允许请求携带的头信息,非简单请求,会进行预检
res.header('Access-Control-Allow-Credentials','true'); // 允许发送cookie,这里前端xhr也需要一起配置 `xhr.withCredentials=true`
next();
});
- 代理
只要是在与你同域下的服务器,新建一个代理(服务端不存在同源策略),将你的跨域请求全部代理转发
后端1
2const proxy=require("http-proxy-middleware"); // 这里使用这个中间件完成代理
app.use('/api', proxy("http://b.com")); // http://a.com/api -> http://b.com/api
- window.name+iframe
MDN里解释道它是获取/设置窗口的名称
,因为的它在不同页面甚至域名加载后值都不会改变,该属性也被用于作为 JSONP 的一个更安全的备选来提供跨域通信(cross-domain messaging)
前端1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21<!--http://a.com/page1.html-->
<script>
function request(url,callback) {
let iframe=document.createElement("iframe");
let isFirst=true;
iframe.style.display="none";
iframe.addEventListener("load",function () {
if (isFirst) {
isFirst=false; // 防止iframe循环加载
iframe.src="http://a.com/page2.html";
callback && callback(iframe.contentWindow.name);
iframe.remove();
}
});
iframe.src=url;
}
requeset("http://b.com/user",function (data) {
console.log(data); // todo
});
</script>
后端1
2
3
4
5
6
7
8
9
10
11
12
13
14// Express(Nodejs)
// mock data
const USERS=[
{name:"Tom",age:23},
{name:"Jack",age:23}
];
app.get("/user",function (req,res) {
res.send(`
<script>
;window.name=${JSON.stringify(USERS)};
</script>
`);
});
document.domian
这个使用情况有限,例如
http://a.c.com
http://b.c.com
主域相同时,分别设置他们页面的document.domain="c.com";
locaction.hash+iframe
嵌套两层iframe,达到第一层与第三层同域,就可以互相通信了
1 | <!--http://a.com/page1.html--> |
1 | <!--http://b.com/user.html--> |
1 | <script> |
- 图片ping
这个只能发出去请求,无法获取到服务器的响应,常常用于网站流量统计
1 | let img=new Image(); |
- postMessage+iframe
1 | <!-- http://a.com --> |
1 | <!-- http://b.com --> |
- postMessage+form+iframe
这个需要后台配合返回特殊格式的数据,TL,DR 可以看这个demo
- WebSocket
WebSocket是一种通信协议,该协议不实行同源政策,
注意需要浏览器和服务器都支持的情况下
1 | <script src="//cdn.bootcss.com/socket.io/1.7.2/socket.io.min.js"></script> |
后端1
2
3
4
5
6
7// Nodejs
const server = require('http').createServer();
const io = require('socket.io')(server);
io.on('connection', function (client) {
client.emit('data', 'This message from "http://b.com"');
});
Summary
- 目前个人在工作中遇到的解决方法就是这些,当然还有许多其他的方法,你看,其实跨域并不难吧 ^_^
- js通过xhr发的跨域请求,虽然得不到响应,但是可以发送出去,其实如果是单向通信的话,也可以,比如文章阅读统计,网站流量统计